《Head First Design Patterns》:第9章,组合(Composite)模式
组合模式的定义
组合模式允许你将对象组合成树形结构来表现“整体/部分”层次结构。组合能让客户以一致的方式处理个别对象以及对象组合,而忽略对象组合和个别对象之间的差异。
场景
紧接迭代器模式,又有咖啡厅被合并进来。
场景描述
咖啡厅被合并进来用来供应晚餐,下面是咖啡菜单:
1 | public class CafeMenu implements Menu { |
依靠已经介绍过的迭代器模式,我们可以很容易的将这个菜单整合进我们的框架,但是我们面临的问题是:每加入一种新菜单就需要改动Waitress
类,并且printMenu(Iterator iterator)
会被每种菜单都调用一次,如下:
1 | public class Waitress { |
并且,餐厅还希望能够加上一份餐后甜点的“子菜单”,这样就像餐厅菜单都会有一个的甜点子菜单。
方案
事情的发展已经到达了一个复杂级别,不可避免地要对菜单进行重新设计了。我们需要:
某种树形结构,可以容纳菜单、子菜单和菜单项;
需要确保我们有一种方法可以遍历到菜单中每个菜单项,至少要像现在迭代器一样方便;
希望能有一个更为灵活的遍历菜单项的方式:比如,我们可能需要仅迭代晚餐的甜点菜单,或者我们可能需要迭代晚餐的整个菜单(包含甜点菜单);
这意味着:如果我们有了一个树形结构的菜单、子菜单和可能还带有菜单项子菜单,那么任何一个菜单都是一种“组合”。
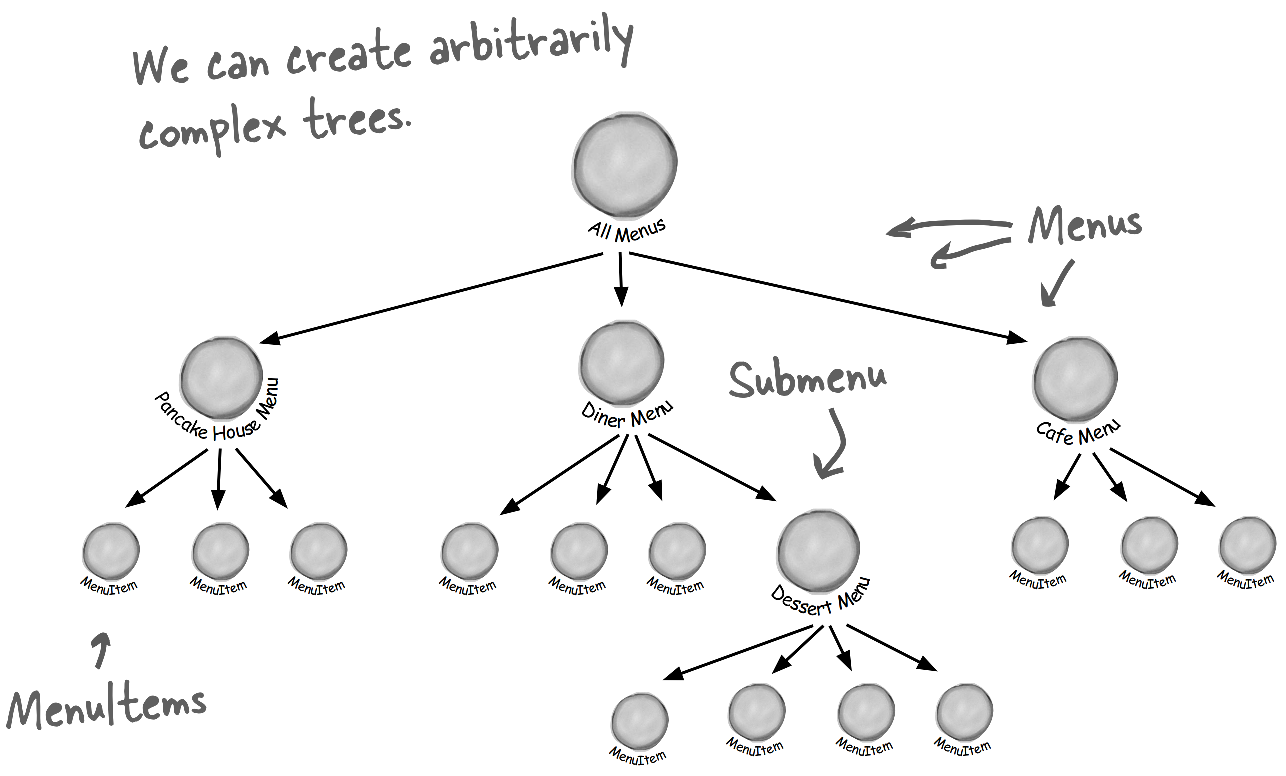
1 | public abstract class MenuComponent { |
1 | public class MenuItem extends MenuComponent { |
1 | public class Menu extends MenuComponent { |
1 | public class Waitress { |
组合迭代器
TODO